Github Pages is a hidden gem that I don’t see many people taking advantage of. It is a great platform to host your projects’ documentation, or any static website for free! I’ll try my best to explain how to use it, and we’ll deploy our first webpage on it.
Table of contents
Open Table of contents
OK, But What Is Github Pages?
Per the official documentation:
GitHub Pages is a static site hosting service that takes HTML, CSS, and JavaScript files straight from a repository on GitHub, optionally runs the files through a build process, and publishes a website.
Or in fewer words, free hosting baby 🚀📈
Should you learn Github Pages?
The short answer? Yes.
The long answer? Yeeeeeeeeesssss.
Hot take Any developer worth their salt should be proficient with the tools they use, or at the very least be aware of the capabilities of said tools, GitHub being one of the most used by more than 100 million of us, knowing more stuff == good, controversial I know.
Anyways, here are some arguments I think will convince you of at least trying it out:
- You won’t get a crippling bill if someone decides to DDoS your TODO react app.
- It’s super simple.
- Costs 0 dollars.
- Native github actions integrations makes deploying with any framework super easy.
- IMO for most websites, PaaS offerings are like using a cannon to kill a fly.
- Your portfolio probably doesn’t need a SLA of 99.9% uptime.
- Without a doubt the best place to host documentation for your projects.
- You clicked on the post already, may as well finish reading it.
I hope my sales pitch was effective and you are already convinced to try it out.
Now my portfolio can handle hundreds of requests per second!!!!1
But now you are probably wondering “how do we use this thing?”.
There’s two schools of thought
FACT: Bears. Eat. Beets.
We have two approaches for deploying on Github Pages:
[0] = The classic way
Github looks for an index.html file in one of the following sources:
- The root of the repository.
- A /docs directory.
It then takes those files and serves them, easy peasy. The default branch is used for deployments (main or master) but we can configure this and deploy from an arbitrary branch (deployments, docs, etc).
The pros
- It’s dead simple, you just push the static files on the configured source and voilà a working website.
- You can specify the branch from where you want to deploy those files easily with a drop-down menu.
The cons
- Very little flexibility.
- You are limited to static files ready to serve, so if you’re using a framework like Astro, for example, you would have to build your webpage beforehand and then commit the built files (do not even attempt to do this, please).
[1] = The DIY way
If you want to have full control of the deployment process you can leverage github actions to achieve this, we will be using this method to build and serve a React app (but you can use whatever shiny framework you like).
The pros
- All the flexibility in the world (thanks to github actions).
- It’s implied in the previous point, but you can bring any framework you like, because we will do the building process and deployment ourselves.
The cons
-
You’ll need to know github actions.
But if you ask me that’s a plus.
I lied about there only being two ways
[2] = The third way
Github actions has a jekyll integration, but I won’t be covering it here, it’s a bit convoluted for my taste and at that point you may as well just use github actions, but yeah well that’s just like my opinion man. You can read about it here.
The boilerplate
First of all I will assume you have a basic understanding of how web frameworks work as well as basic git and github for this section (You know how to git push to a remote repository). You can take a look at the finished repo here
1. Setup the repo
Go and create a repository on github and name it whatever you like, in my case I will name it github-pages-example:
2. Scaffold the project
On your local machine let’s create the react project using vite:
npm create vite@latest
Which vite options you choose here are irrelevant for the purposes of the tutorial, but you should end up with something like this:
Now inside the repo let’s install our dependencies and run the project to verify everything is a-ok:
npm i
npm run dev
Go to the localhost url (usually localhost:4321) and you should see the vite starting page:
Now let’s create a .github
directory with a workflows
directory inside,
here’s were our workflow files will live:
mkdir -p .github/workflows
And that’s it, that’s all the boilerplate we need to start working:
.
├── eslint.config.js
├── .github
│ └── workflows
├── .gitignore
├── index.html
├── package.json
├── package-lock.json
├── public
│ └── vite.svg
├── README.md
├── src
│ ├── App.css
│ ├── App.tsx
│ ├── assets
│ │ └── react.svg
│ ├── index.css
│ ├── main.tsx
│ └── vite-env.d.ts
├── tsconfig.app.json
├── tsconfig.json
├── tsconfig.node.json
└── vite.config.ts
6 directories, 17 files
You should end up with something similar
3. Vite specific config
The base default path for vite is /
but our deployment url will look something
like this: https://<USERNAME>.github.io/<REPO>/
. Leaving this base path will
means the browser will try to fetch static files from
https://<USERNAME>.github.io/style.css
instead of
https://<USERNAME>.github.io/<REPO>/style.css
, for example, leading to a 404.
Read more about url paths
here.
Having explained the why, let’s now configure the base
property in the
configuration file (vite.config.ts
in my case) to use the name of our repo, in
my case /github-pages-example/
:
We could also use a relative path "./"
but vite recommends using the repo name
on their docs.
4. Push to remote
Hit it with some good ol’ git action:
git init -b main
git add -A
git commit -m "First commit"
git remote add origin git@github.com:0bCdian/github-pages-example.git
git push -u origin main
And now you should see the remote repo with the recent changes reflected:
5. Configuring the deployment source
In the github repo go to settings > pages:
Click the gear icon
Click the pages tab
Now in the pages tab we’re going to change the source from branch to github action:
Before
After
And that’s all the configuring we need for now. Next on the list is creating the workflow file that will build and deploy our site.
But first…
Github actions crash course
If you’re already familiar with github actions feel free to skip ahead, for everyone else here’s the quick and dirty explanation:
Github actions is a CI/CD platform for automating your workflows, without
getting into much detail, it boils down to writing configuration files that
define the steps we want to execute every time something happens (we decide
which something). The files are written in YAML and must be
created inside the .github/workflows
directory. When a new commit is pushed,
github looks in the workflows directory for files, parses them and if the
triggers are met, the workflows run. If you don’t even know yaml/yml do not
fret, it’s just a configuration language that uses indentation for specifying
context similar to python, and you can learn the gist of it in like
5 minutes, if you’d like a more thorough
explanation
go read the friendly manual here.
Overly simplified diagram of github actions
When you create your workflows and push them to a branch they will be picked up by github and will appear in the actions tab of your repository, like so:
The guts of a workflow
I will be very brief about the syntax but here’s the usual structure goes something like this:
# Optional name for the workflow, this name is what shows up in the actions tab
name: GitHub Actions Demo
# The name that will appear in the "black box" (the workflow instance if you will)
run-name: ${{ github.actor }} is testing out GitHub Actions 🚀
# Definitions of events that trigger this workflow
on:
# For example, on pushes...
push:
# In the main branch
branches: [main]
# The list of jobs this workflow will run
jobs:
# The name of the job
Explore-GitHub-Actions:
# The machine that will run this job (the os basically)
runs-on: ubuntu-latest
# The steps of this job
steps:
# steps run commands in the shell (bash in this case)
# They can have names, in this case the first three don't
- run: echo "🎉 The job was automatically triggered by a ${{ github.event_name }} event."
- run: echo "🐧 This job is now running on a ${{ runner.os }} server hosted by GitHub!"
- run: echo "🔎 The name of your branch is ${{ github.ref }} and your repository is ${{ github.repository }}."
# This named step "uses" the action "action/checkout" version 4
- name: Check out repository code
uses: actions/checkout@v4
# More unnamed steps
- run: echo "💡 The ${{ github.repository }} repository has been cloned to the runner."
- run: echo "🖥️ The workflow is now ready to test your code on the runner."
# Another named step
- name: List files in the repository
run: |
ls ${{ github.workspace }}
# You get the idea
- run: echo "🍏 This job's status is ${{ job.status }}."
There’s a lot to unpack here but the general structure of a workflow is:
- The name of the workflow (optional)
- The name of the workflow instance, or run-name (optional)
- The triggers that kick off the workflow, defined with the
on
keyword. - The jobs in the workflow.
- The steps in a job.
In short a workflow is a series of jobs, a job is a series of steps.
You probably noticed that we used two different keywords in the steps, uses
and run
:
run
is for running commands directly in the shell of the operating system (bash, powershell, etc).uses
is meant for running actions.
And what is an action then? You may ask
You can think of an action as a song, and a workflow as a playlist. In more
technical terms, an action is a self-contained piece of code meant to be run as
a step in a larger workflow. There’s actually a
marketplace for actions and
Github itself maintains quite a lot of them. The
name of an action has the following structure repo/action-name@version
, for
example, actions/checkout@v4
which clones the repo, and then configures the
working directory of the machine to be the root of your repository (I would bet
this is the most used action by far).
I won’t extend further as I could write a blog just on this topic, refer to the docs if you want to learn more about it.
Running our first workflow
Let’s paste the example workflow from the
the previous section in a file called
my-first-action.yml
inside the .github/workflows/
directory:
cat <<EOF >>.github/workflows/my-first-action.yml
name: GitHub Actions Demo
run-name: ${{ github.actor }} is testing out GitHub Actions 🚀
on:
push:
# In the main branch
branches: [main]
jobs:
Explore-GitHub-Actions:
runs-on: ubuntu-latest
steps:
- run: echo "🎉 The job was automatically triggered by a ${{ github.event_name }} event."
- run: echo "🐧 This job is now running on a ${{ runner.os }} server hosted by GitHub!"
- run: echo "🔎 The name of your branch is ${{ github.ref }} and your repository is ${{ github.repository }}."
- name: Check out repository code
uses: actions/checkout@v4
- run: echo "💡 The ${{ github.repository }} repository has been cloned to the runner."
- run: echo "🖥️ The workflow is now ready to test your code on the runner."
- name: List files in the repository
run: |
ls ${{ github.workspace }}
- run: echo "🍏 This job's status is ${{ job.status }}."
EOF
One-liner bash command to create the workflow
Then commit this file and push it to the remote:
git add .github/workflows/my-first-action.yml
git commit -m "Adding our first workflow!"
git push origin main
You will see a yellow dot beside the commit message, and on the actions tab as
well, this means the workflow was read successfully and is currently running:
Navigate to the actions tab and you should see the worfklow in action:
Inspecting a job
Congratulations, you just ran your first workflow! 🎉
There’s a lot more you can do with workflows, but I can’t cover all of it in this post for brevity’s sake, go to the official documentation to learn more about github action workflows.
Now let’s get down to business.
4..3..2..1.. Deploy!! 🚀
Now back on our local repo, let’s write the workflow for deploying our site.
This is the basic overview of what we are going to do:
If you check your package.json
you will see a build command, if you run it
vite will output a ./dist
directory with the contents of the website ready to
serve. We are going to upload those files to an
artifact
and deploy them with the help of these two official github actions:
The names are self-explanatory but the general idea is that the first one takes the directory we give it as an input, and packages it in a specific way before uploading it as an artifact. The second one downloads the artifact we uploaded before, and creates a new deployment with the static files downloaded.
We need to make a quick stop on environments
Environments are used to describe a general deployment target like production, staging, or development. When a GitHub Actions workflow deploys to an environment, the environment is displayed on the main page of the repository.
The reason we need them for using Github Pages is because:
But really, the reason lies within the capabilities that environments give you, like branch protection rules, variables and secrets managing, etc, so github by default requires that all deployments sit on top of an environment, and since we are going to make a deployment, we therefore need an environment.
Back on track
Let’s create a file called deploy.yml
(the name is arbitrary) and open it in
your favorite editor, bonus points if it’s neovim (I use neovim btw):
This is what we are going to paste in that file:
name: Deploy to github pages
on:
# on pushes...
push:
# to the main branch...
branches: ["main"]
paths: ["src/**"]
# on workflow_dispatch enables a button to run this action manually
workflow_dispatch:
jobs:
build:
runs-on: "ubuntu-latest"
steps:
# first step git clone the repo
- name: Checkout code
uses: actions/checkout@v4
# set up nodejs in the ubuntu machine
- name: Setup node
uses: actions/setup-node@v4
with:
node-version: 22
# install dependencies (npm ci ensures the package-lock is not modified)
- name: Install deps
run: |
npm ci
# we then build our website, default destination of our static files is
# ./dist
- name: Build
run: |
npm run build
# we take the ./dist dir and upload it an artifact
- name: Upload static files to an artifact
id: build
if: ${{success()}}
uses: actions/upload-pages-artifact@v3
with:
path: "./dist"
# we kick the second job called deploy
deploy:
# this establishes a dependency between the previous job and this one
# so if the previous fails, this ones doesn't executes
needs: build
# we specify the permissions needed for the next step, granted to this runner
permissions:
pages: write
id-token: write
# we specify the environment for this deployment, called github-pages
environment:
name: github-pages
# we set the environment url to the output of the next step
# this may confuse you but this runs asynchronously
# even though we are defining a field before the step is run and has
# output anything yet
url: ${{ steps.deployment.outputs.page_url }}
runs-on: "ubuntu-latest"
steps:
# We kick off the deployment
- name: Deploy to github pages
id: deployment
uses: actions/deploy-pages@v4
After that some git elbow grease:
git add .github/workflows/deploy.yml
git commit -m "Let's deploy some pages!"
git push origin main
And then check your actions tab to witness the magic happening 🪄:
🦗🦗🦗
Absolutely nothing happened:
You’ve been bamboozled
Don’t worry, if you’ve been following the tutorial step by step I did this on purpose.
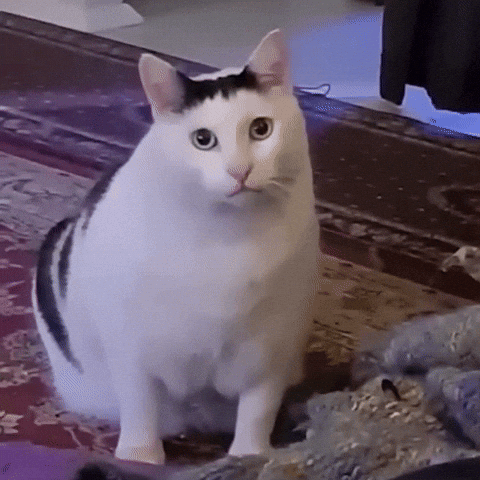
If you paid attention, in the trigger we specified a path
condition, so not
only do we have to push to main, we also have to push changes to /src/**
specifically for the workflow to run.
name: Deploy to github pages
on:
push:
branches: ["main"]
# Here's the ඞ
paths: ["src/**"]
So let’s do just that, edit the src/App.tsx
file and add any changes you like:
Commit and push those changes and you should see the deploy workflow running (I swear):
Click on the link aaaaand:
+500 Aura
You’ve reached the end of the post traveler, feel free to rest here.
Conclusion
Congratulations! You’re now the proud recipient of -20 minutes of your life. 😆 Jokes aside, I hope you learned something new and can apply this knowledge to other frameworks.
If you found this post helpful or have any thoughts to share, feel free to reach out to me on social media. Thanks for reading! ✌🏾